How to get started with 3LC Python package#
3LC consists of the Dashboard and the 3LC Python package. To use 3LC, you probably want to start with the 3LC Python package and create some content to be viewed in the Dashboard, unless you just want to view some existing projects in the Dashboard.
Did you know?
You can view some pre-populated projects in our Demo Dashboard without installing the 3LC Python package. It’s a good place to dip your toe in the water before you commit to 3LC.
On this page, we introduce some basic methods and help you create your first project and 3LC Table.
Assuming that you have installed the 3LC Python package (How to install 3LC), the first step is almost always:
import tlc
If you are wondering why it is called tlc
instead of 3lc
, here is the answer.
Let’s use the API to create a Table. A Table is a basic data structure in 3LC that stores tabular data. We use Tables to store data including images and labels, etc. But, a Table can be as simple as the example below.
table = tlc.Table.from_dict(
{"name": ["Adam","Lily", "Zack"], "pass": [True,True,False], "score": [90,75,50]},
project_name="my_project",
dataset_name="my_dataset",
table_name="my_table")
Note that a dataset is one level above the Table, because a dataset can have multiple Tables. A new Table or revision, which are interchangeable, will be created when you edit your data and commit it. Both the original Table and edited Table belong to the same dataset. For more in-depth information about creating a Table, please see how to create a Table.
When you create a Table, it is highly recommended to specify a project name as shown in the example above, even though doing so is optional. If it does not already exist, the project folder will be automatically created to contain the Table.
Once you run the code above, the project and Table will be created and stored in the 3LC default directory. For the default directories for different operating systems, please see Default File Locations. Meanwhile, you will be able to see the project and the Table in the Dashboard if the 3LC object service is running (how to start the 3LC object service). This page gives you a quick guide on how to open a project in the Dashboard.
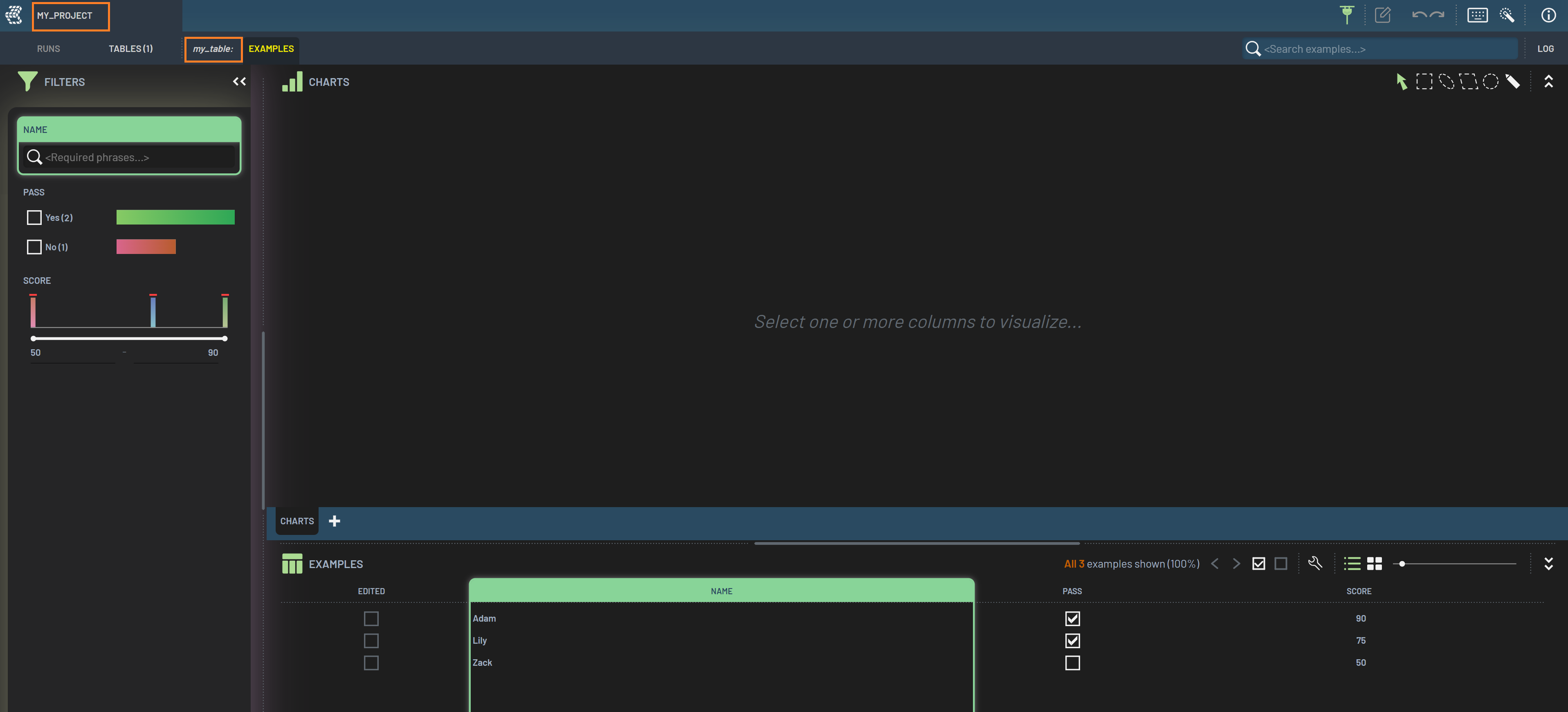
Next, let’s look into some properties of the Table.
# Get Table's url
table.url
# Url('C:/Users/<your name>/AppData/Local/3LC/3LC/projects/my_project/datasets/my_dataset/tables/my_table')
# Get Table's name
table.name
# 'my_table'
# Return the number of rows in the Table
len(table)
# 3
# Get Table's column names
table.columns
# ['name', 'pass', 'score', 'weight']
# Note: "weight" is an automatically added column when creating a Table
# Return the first row of the Table
table[0]
# {'name': 'Adam', 'pass': True, 'score': 90}
# Note: this way does not return "weight" and its value.
# Return the first row of the Table
table.table_rows[0]
# ImmutableDict({'name': 'Adam', 'pass': True, 'score': 90, 'weight': 1.0})
# Note: this way returns "weight" and its value
# Output each row's content
for row in table:
print(row)
# {'name': 'Adam', 'pass': True, 'score': 90}
# {'name': 'Lily', 'pass': True, 'score': 75}
# {'name': 'Zack', 'pass': False, 'score': 50}
To get an existing Table:
# Get a Table from URL
table = tlc.Table.from_url('path/to/table')
# Note: A Table is a folder consisting of a json file and a parquet file or just a json file,
# so a Table's URL is the path to the folder.
# Get a Table from names
table = tlc.Table.from_names(
table_name = "my_table",
dataset_name = "my_dataset",
project_name = "my_project"
)
When multiple revisions exist in a dataset, the method latest()
can be used to get the latest revision.
table = table.latest()